Development of a tool to send static mesh and ISMs from Maya to Unreal. Once it's complete I'll move the finished code up top for easy answer in future.
Maya side python code so far (by the way, code tags don't seem to format properly for me):
import maya.cmds as cmds
import csv
selection = cmds.ls(sl=True)
col = ['','actorName','tx', 'ty', 'tz', 'rx', 'ry', 'rz', 'sx', 'sy', 'sz']
with open("C:\ISMExporter\data.csv", 'w') as f:
writer = csv.writer(f)
writer.writerow(col)
for idx, obj in enumerate(selection):
data = []
data.append(idx)
data.append(idx)
actorName = obj.split("_")[0]
data.append(actorName)
data.append(cmds.getAttr(obj + '.translateX'))
data.append(cmds.getAttr(obj + '.translateY'))
data.append(cmds.getAttr(obj + '.translateZ'))
data.append(cmds.getAttr(obj + '.rotateX'))
data.append(cmds.getAttr(obj + '.rotateY'))
data.append(cmds.getAttr(obj + '.rotateZ'))
data.append(cmds.getAttr(obj + '.scaleX'))
data.append(cmds.getAttr(obj + '.scaleY'))
data.append(cmds.getAttr(obj + '.scaleZ'))
writer.writerow(data)
del data
Trying to figure out where the mystery rows come from.
This is only basic test to figure out how we can get .uassets from content browser by name. This proves that it can be done and the asset loaded from construction script. Next I will need to parse the data table, but is easy. The part I'm still unsure about is how to handle reimport, but just going one problem at a time.
Replies
import csv
selection = cmds.ls(sl=True)
col = ['','actorName','tx', 'ty', 'tz', 'rx', 'ry', 'rz', 'sx', 'sy', 'sz']
with open("C:\ISMExporter\data.csv", 'w', newline='') as f:
writer = csv.writer(f)
writer.writerow(col)
for idx, obj in enumerate(selection):
data = []
data.append(idx)
actorName = obj.split("_")[0]
data.append(actorName)
data.append(cmds.getAttr(obj + '.translateX'))
data.append(cmds.getAttr(obj + '.translateY'))
data.append(cmds.getAttr(obj + '.translateZ'))
data.append(cmds.getAttr(obj + '.rotateX'))
data.append(cmds.getAttr(obj + '.rotateY'))
data.append(cmds.getAttr(obj + '.rotateZ'))
data.append(cmds.getAttr(obj + '.scaleX'))
data.append(cmds.getAttr(obj + '.scaleY'))
data.append(cmds.getAttr(obj + '.scaleZ'))
writer.writerow(data)
del data
In unreal, the only additional thing to do is make the Data Table an instance editable variable, this way you can have each one of these "ISM Loader" actors represent some export group. Again, that can be an individual model, or a whole neighborhood, or even an entire scene if it wasn't too large. It's just a balance of how many individual exports you want to make, versus how granular your iterative changes are likely to be.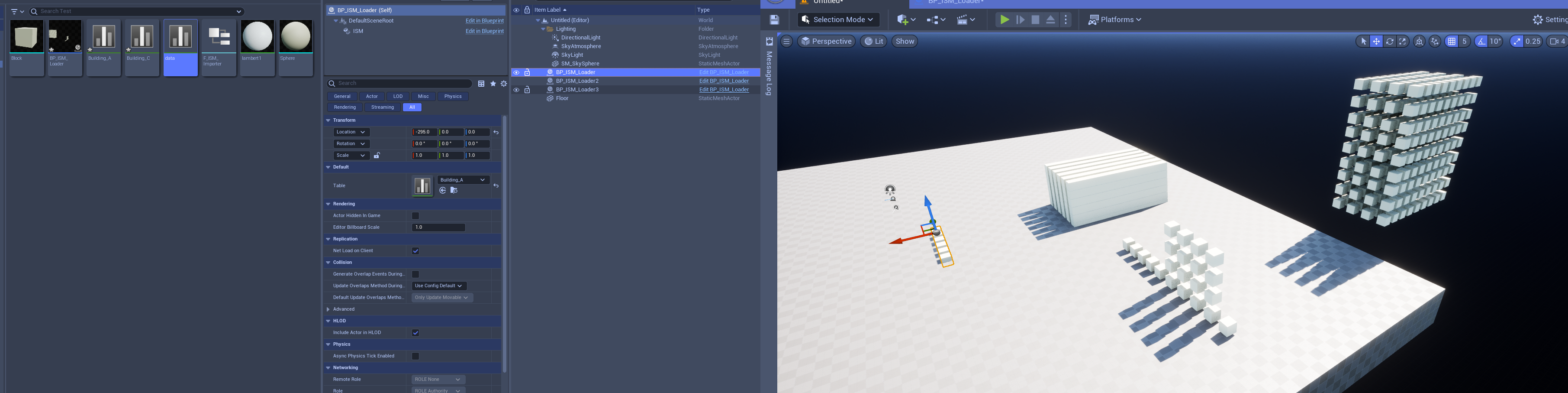
So the workflow is pretty simple: