I can't figure out why I am only able to spawn 1 object, it correctly spawns 10 clones, but I am only able to activate one of them at any given time. Does anyone know why this is?
Script A:
using UnityEngine;
using System.Collections.Generic;
public class Pool : MonoBehaviour
{
public GameObject[] objects;
public int[] number;
public List<GameObject>[] pool;
// Use this for initialization
void Start ()
{
instantiate ();
}
// Instantiate all the objects
void instantiate()
{
GameObject temp;
pool = new List<GameObject>[ objects.Length ];
for ( int count = 0; count < objects.Length; count++ )
{
pool[ count ] = new List<GameObject>();
for( int num = 0; num < number[ count ]; num++ )
{
temp = ( GameObject ) Instantiate( objects[ count ] );
temp.transform.parent = this.transform;
pool[ count ].Add ( temp );
}
}
}
public GameObject activate( int id )
{
for( int count = 0; count < pool[ id ].Count; count++ )
{
if( !pool[ id ][ count ].activeSelf );
{
pool[ id ][ count ].SetActive ( true );
return pool[ id ][ count ];
}
}
pool [ id ].Add ( ( GameObject ) Instantiate ( objects[ id ] ) );
pool[ id ][ pool[ id ].Count - 1 ].transform.parent = this.transform;
return pool[ id ][ pool[ id ].Count - 1 ];
}
public GameObject activate( int id, Vector3 position, Quaternion rotation )
{
for( int count = 0; count < pool[ id ].Count; count++ )
{
if( !pool[ id ][ count ].activeSelf );
{
pool[ id ][ count ].SetActive ( true );
pool[ id ][ count ].transform.position = position;
pool[ id ][ count ].transform.rotation = rotation;
return pool[ id ][ count ];
}
}
//I may need to fix this so it doesn't spawn at the last known location
pool[ id ].Add( ( GameObject ) Instantiate( objects[ id ] ) );
pool[ id ][ pool[ id ].Count - 1 ].transform.position = position;
pool[ id ][ pool[ id ].Count - 1 ].transform.rotation = rotation;
pool[ id ][ pool[ id ].Count - 1 ].transform.parent = this.transform;
return pool[ id ][ pool[ id ].Count - 1 ];
}
public void deActivate( GameObject deActivateObject )
{
deActivateObject.SetActive ( false );
}
}
Script B: just to test the spawning
using UnityEngine;
using System.Collections;
public class Shoot : MonoBehaviour {
public Pool pool;
// Use this for initialization
void Start ()
{
}
// Update is called once per frame
void Update ()
{
if( Input.GetKeyDown( KeyCode.C ) )
{
pool.activate( 0, new Vector3( 1.3f, 0f, 0.1f), Quaternion.identity );
}
// If I have a second Element I want to add
// else if( Input.GetKeyDown( KeyCode.S ) )
// {
// pool.activate( 1, , new Vector3( 1.3f, 0f, 0.1f), Quaternion.identity );
// }
}
}
Script C: attached to the prefab object (the sphere in the PoolingObjects folder)
using UnityEngine;
using System.Collections;
public class PoolMember : MonoBehaviour
{
public float life;
float timeToDie;
// Use this for initialization
void OnEnable ()
{
timeToDie = life + Time.time;
}
// Update is called once per frame
void Update ()
{
if( Time.time > timeToDie )
{
gameObject.SetActive ( false );
}
}
}
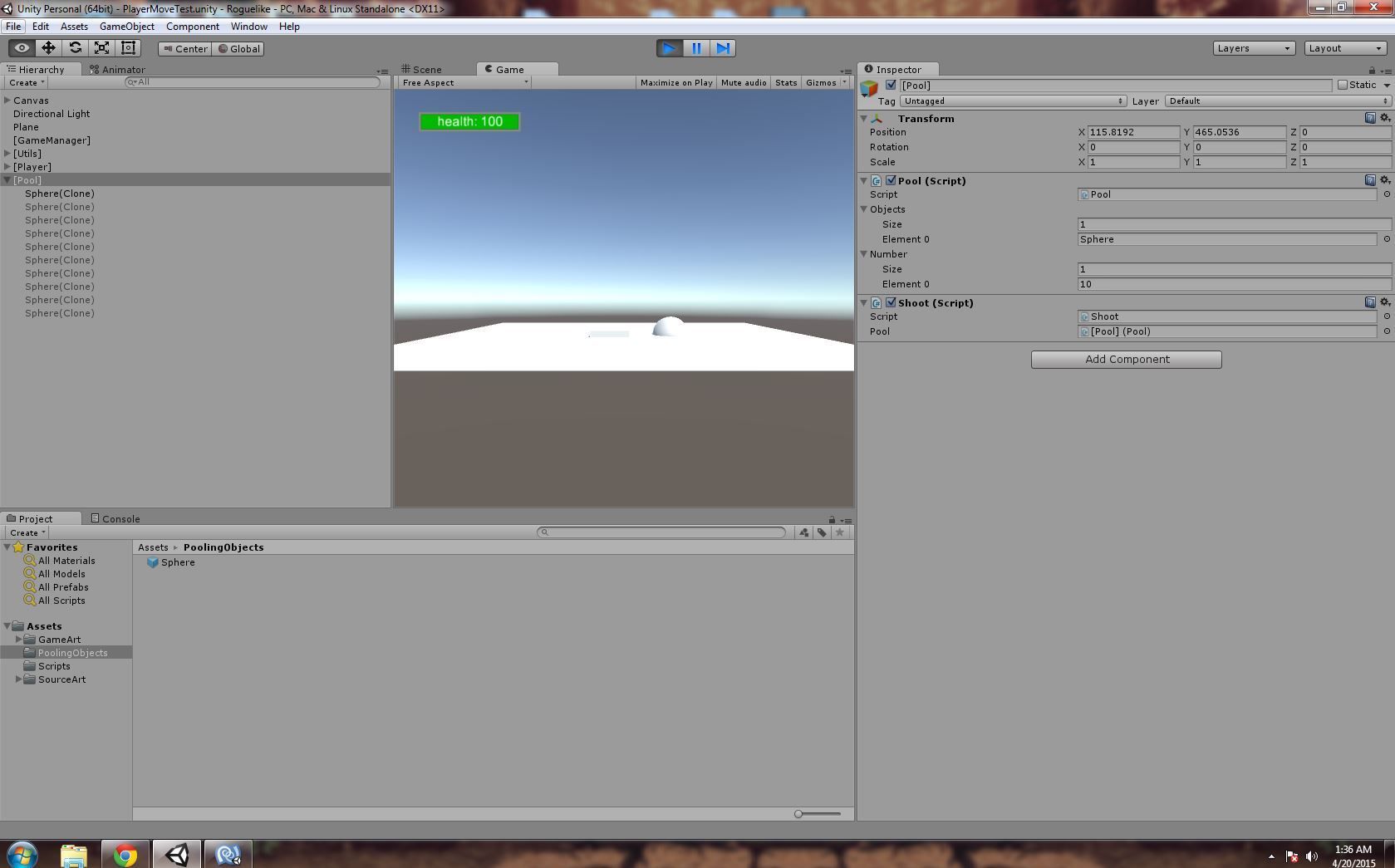
The Sphere in PoolingObjects is the prefab being instantiated (Element 0 ).
For some reason only one clone is able to become active when I press the C key.
Replies
In my example you will soon run out of objects on the list though. So you could check if it has reached the end of the list and then just reset the varianle to zero: