Hey everybody I'm just trying to have my bullet destroy on impact. My bullet is a prefab. Here is my unity screen
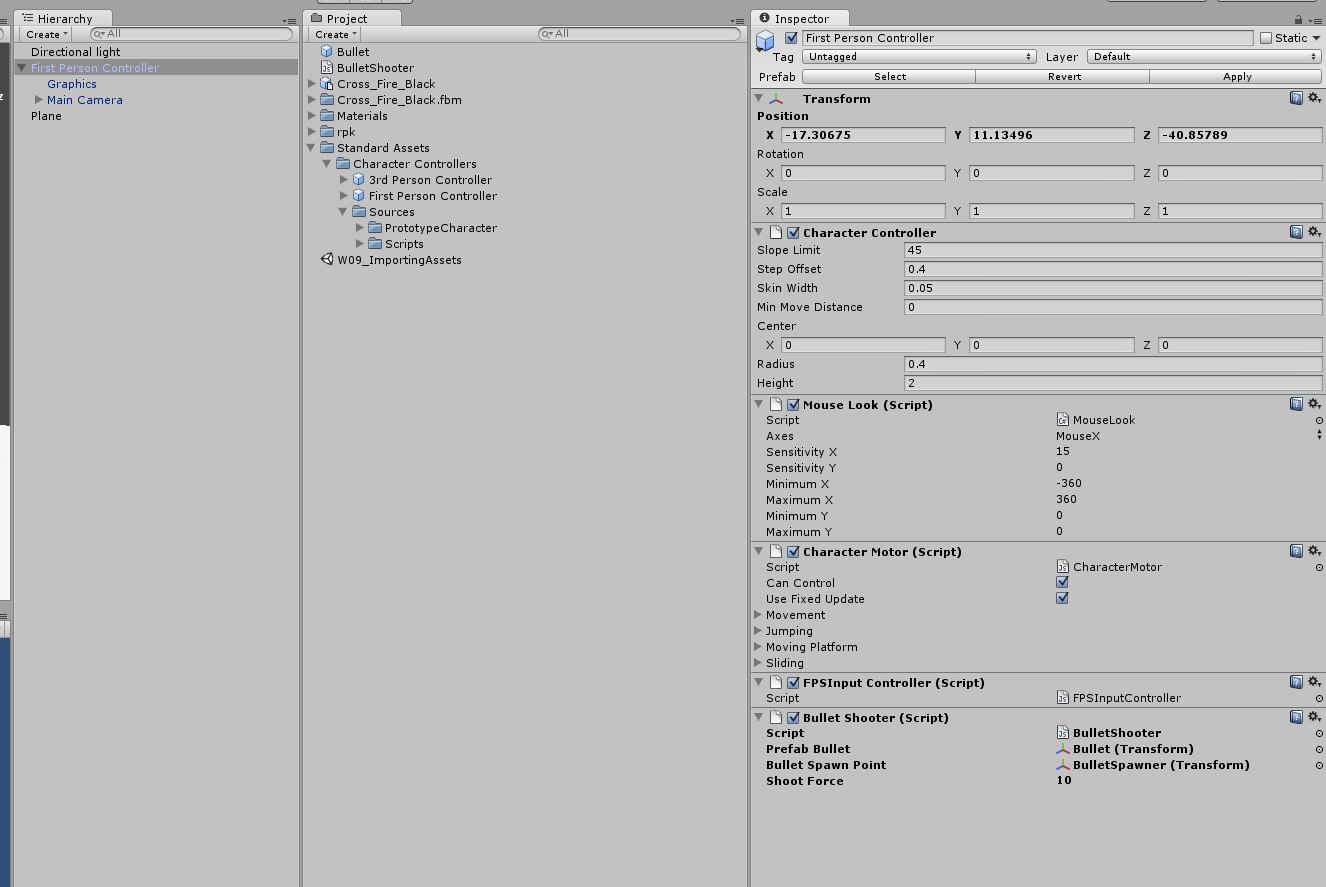
Here is my code:
public var prefabBullet:Transform; //object to create
public var bulletSpawnPoint:Transform; //place to create object
public var shootForce:float; //speed of object
function Start () {
}
function Update ()
{
if(Input.GetButton("Fire1")) //if left click is pressed
{
//create the bullet
var bulletInstance = Instantiate(prefabBullet,bulletSpawnPoint.position, bulletSpawnPoint.rotation);
//fire the bullet
bulletInstance.rigidbody.AddForce(bulletSpawnPoint.transform.forward*shootForce);
}
}
function OnCollisionEnter(c:Collision)
{
if(c.gameObject.name == "Bullet")
{
Destroy(c.gameObject);
}
}
Every time I shoot the plane it just bounces off! What can I do????
Replies
The OnCollisionEnter function is called when whatever the script is attached to collides. If you want that function called when the bullet collides, you need that script attached to the bullet.
Create a new script with that function, and attach it to the bullet prefab.
and I attached my code to the gameobject
Here is my code:
#pragma strict
public var prefabBullet:Transform; //object to create
public var bulletSpawnPoint:Transform; //place to create object
public var shootForce:float; //speed of object
function Start ()
{
}
function Update ()
{
if(Input.GetButton("Fire1")) //if left click is pressed
//create the bullet
var bulletInstance = Instantiate(prefabBullet,bulletSpawnPoint.position , bulletSpawnPoint.rotation);
//fire the bullet
bulletInstance.rigidbody.AddForce(bulletSpawnPoint .transform.forward*shootForce);
}
function OnCollisionEnter(c:Collision)
{
if(c.gameObject.name == "Bullet_Prefab")
{
Destroy(c.gameObject);
}
}
The Bullet still won't disappear and I get errors, here they are:
NullReferenceException: Object reference not set to an instance of an object
BulletCollide.Update () (at Assets/BulletCollide.js:19)
instead, keep the bullet shooting script on the player as it was in your original post, but remove the OnCollisionEnter function from that script.
then create a new script that is only applied to the bullet prefab and give it like
function OnCollisionEnter ()
{
Destroy(gameObject);
}
don't put this new script on the player. put it only on the bullet prefab which gets instantiated by the player's shooting script.
be warned that the bullet might delete itself instantly if you spawn it inside another collider, like the player's.
function Start ()
{
}
function Update ()
{
}
function OnCollisionEnter(c:Collision)
{
if(c.gameObject.name == "Bullet_Prefab")
{
Destroy(c.gameObject);
}
}
But it still won't destroy and I can't figure it out
Delete this part, because it doesn't do what you are looking for. The OnCollosionEnter function does not return the collider of your bullet. It returns the collider of the object that the object with the attached script just entered!
function Start ()
{
}
function Update ()
{
}
function OnCollisionEnter(c:Collision)
{
Destroy(c.gameObject);
}
But whatever the bullet touches it destroys. For ex: I shoot cube, cube gets destroyed. What would I write so that bullet gets destroyed if it collides?
function OnCollisionEnter()
{
Destroy (gameObject);
}
don't forget to add collider to your bullet for that snipet to work.. If your bullets move too quickly it could be worth to expand shooting script with raycasting to actualy get impact point in advance and calculate timed destruction in advance..
You're having way too many problems with way too basic of a script and you seem to be blindly doing what we say rather than understanding what we're telling you. So sit yourself down, and learn to program. If you're doing this to learn to program then quite carefully listen to the following.
DEBUGGING: This is where you analyze what your code is doing versus what you thought it should do. If you would have added a breakpoint or logged some info as to what the system was doing during each of those steps you would have seen what was happening and learnt how to fix it for yourself. Programming is about figuring out how to get something done with the tools at your disposal if you don't stop and evaluate your tools and think how to apply them to solving your situation then you're going to have a bad time.
So let's analyze your time problem, well how does time pass? Maybe there's a Timer class to track time for me, but where would I put it? Hey that update function is called every tick, so lets put it in there. I don't need a new timer every tick so I'll create it in the Start function. Now during the tick function I can ask it if it's met it's time already and if it has, I can re-use the code from my Destroy to get rid of it! But wait, what if the bullet gets destroyed before I run out of time? I guess I should remove the timer in my destroy function.
And there's your answer. I won't give that to you in code because I think you should be able to figure it out from that and if you can't then it's a learning experience you need.
Hope you'll get serious about learning to program
The Unity Online Reference is extensive and documents almost everything ,there are the few obscure things that are not documented well, but it works.
Object Destroy - Link.
Programming does take a certain mindset, I know I find it hard to switch from doing art to doing code after a hiatus. But no matter what, learning how to dig through the documentation will help tons.
Post your progress. Pass your class. If you want to get into programming even a little, take it in small bites.
- My favorite quote from my friend.
Needs to learn to program. That if statement lacks curly braces, so it's only gonna grab the next line which means it's going to suck up the variable declaration. Now, depending upon your language that may just be an assignment but either way when the next line after that access a parameter of a potentially null variable without checking... you've got problems
It's why I wrote it as a more general "Learn to Program" than "Read your docs" because I saw more than one problem in the whole chain of events.
2. Add it to your bullet prefab
Change this to this if you do not pre-cache bullets.